Reference Compositions
This page presents the range of compositions within the reference compositon database
accessible within pyrolite
. It’s currently a work in progress, but will soon
contain extended descriptions and notes for some of the compositions and associated
references.
import matplotlib.pyplot as plt
from pyrolite.geochem.norm import all_reference_compositions, get_reference_composition
refcomps = all_reference_compositions()
norm = "Chondrite_PON" # a constant composition to normalise to
Chondrites
fltr = lambda c: c.reservoir == "Chondrite"
compositions = [x for (name, x) in refcomps.items() if fltr(x)]
fig, ax = plt.subplots(1)
for composition in compositions:
composition.set_units("ppm")
df = composition.comp.pyrochem.normalize_to(norm, units="ppm")
df.pyroplot.REE(unity_line=True, ax=ax, label=composition.name)
ax.legend()
plt.show()
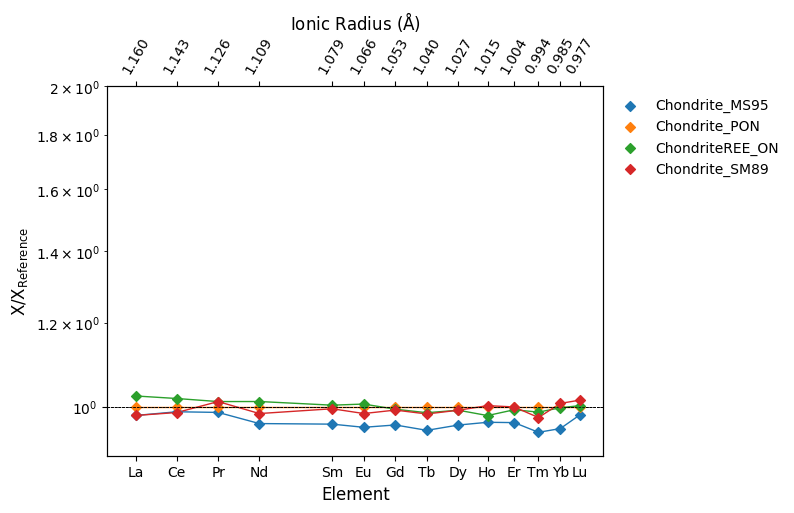
Mantle
Primitive Mantle & Pyrolite
fltr = lambda c: c.reservoir in ["PrimitiveMantle", "BSE"]
compositions = [x for (name, x) in refcomps.items() if fltr(x)]
fig, ax = plt.subplots(1)
for composition in compositions:
composition.set_units("ppm")
df = composition.comp.pyrochem.normalize_to(norm, units="ppm")
df.pyroplot.REE(unity_line=True, ax=ax, label=composition.name)
ax.legend()
plt.show()
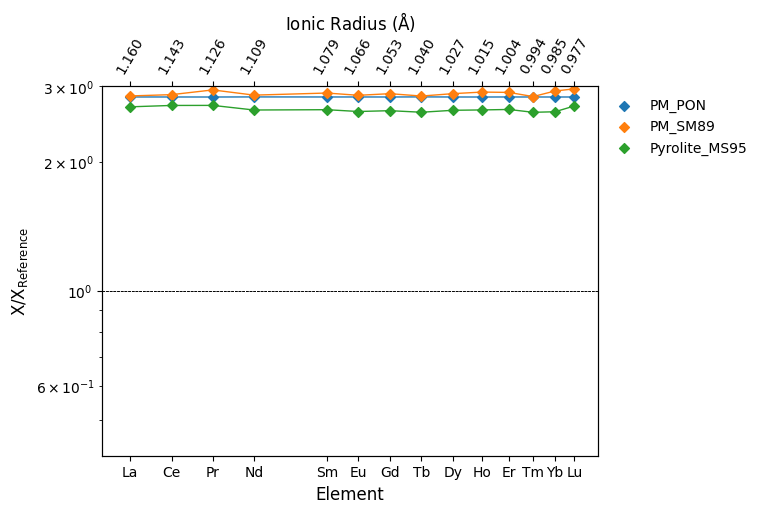
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
Depleted Mantle
fltr = lambda c: ("Depleted" in c.reservoir) & ("Mantle" in c.reservoir)
compositions = [x for (name, x) in refcomps.items() if fltr(x)]
fig, ax = plt.subplots(1)
for composition in compositions:
composition.set_units("ppm")
df = composition.comp.pyrochem.normalize_to(norm, units="ppm")
df.pyroplot.REE(unity_line=True, ax=ax, label=composition.name)
ax.legend()
plt.show()
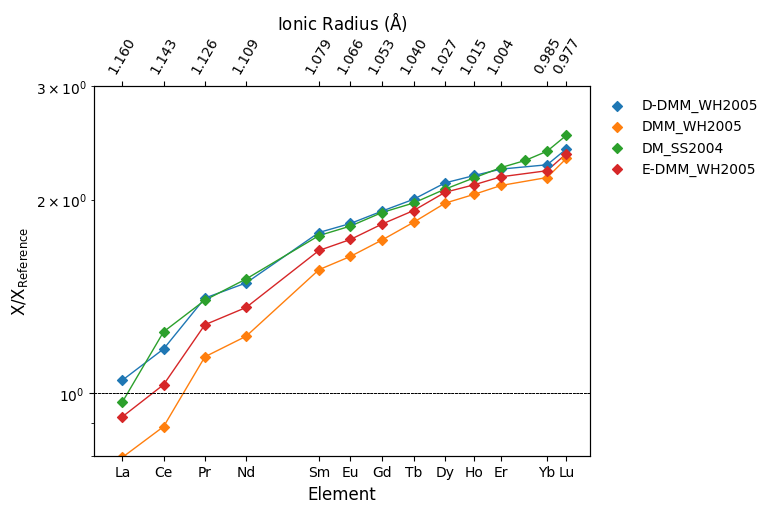
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
Mid-Ocean Ridge Basalts (MORB)
Average MORB, NMORB
fltr = lambda c: c.reservoir in ["MORB", "NMORB"]
compositions = [x for (name, x) in refcomps.items() if fltr(x)]
fig, ax = plt.subplots(1)
for composition in compositions:
composition.set_units("ppm")
df = composition.comp.pyrochem.normalize_to(norm, units="ppm")
df.pyroplot.REE(unity_line=True, ax=ax, label=composition.name)
ax.legend()
plt.show()
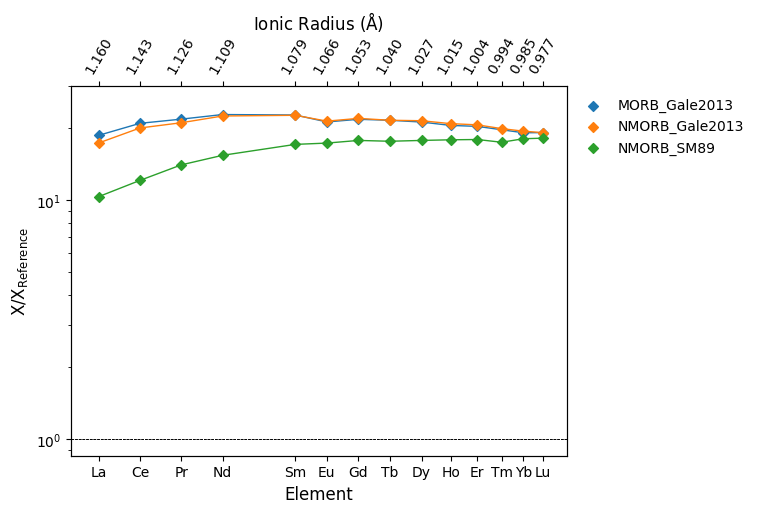
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
Enriched MORB
fltr = lambda c: "EMORB" in c.reservoir
compositions = [x for (name, x) in refcomps.items() if fltr(x)]
fig, ax = plt.subplots(1)
for composition in compositions:
composition.set_units("ppm")
df = composition.comp.pyrochem.normalize_to(norm, units="ppm")
df.pyroplot.REE(unity_line=True, ax=ax, label=composition.name)
ax.legend()
plt.show()
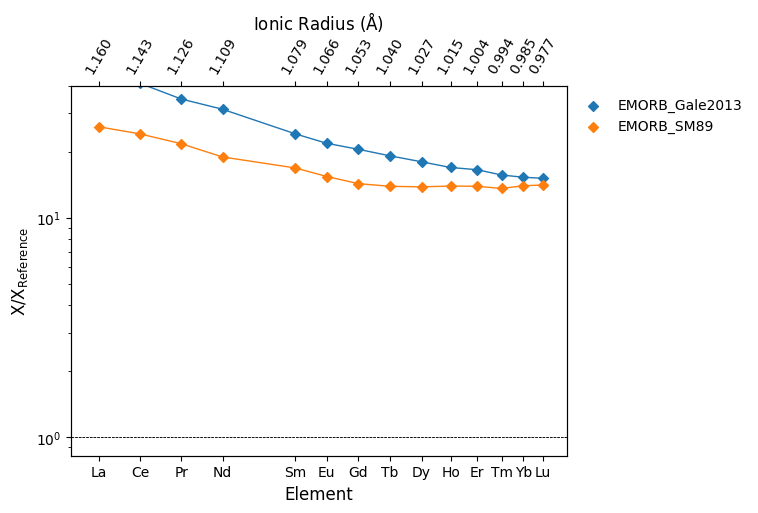
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
Ocean Island Basalts
fltr = lambda c: "OIB" in c.reservoir
compositions = [x for (name, x) in refcomps.items() if fltr(x)]
fig, ax = plt.subplots(1)
for composition in compositions:
composition.set_units("ppm")
df = composition.comp.pyrochem.normalize_to(norm, units="ppm")
df.pyroplot.REE(unity_line=True, ax=ax, label=composition.name)
ax.legend()
plt.show()
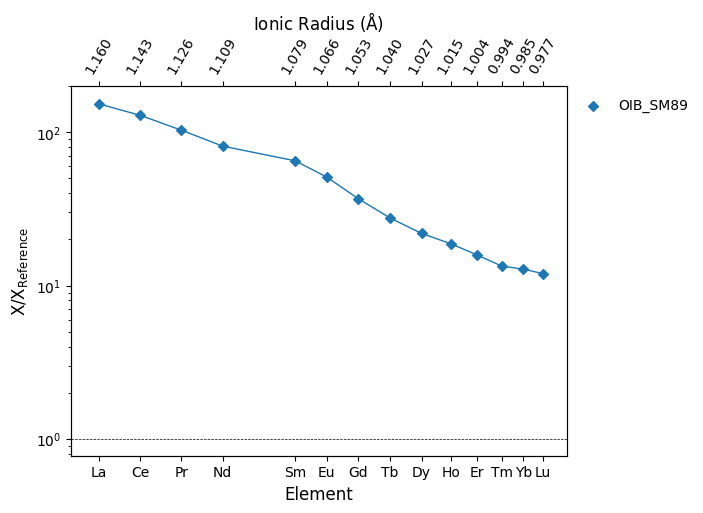
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
Continental Crust
Bulk Continental Crust
fltr = lambda c: c.reservoir == "BulkContinentalCrust"
compositions = [x for (name, x) in refcomps.items() if fltr(x)]
fig, ax = plt.subplots(1)
for composition in compositions:
composition.set_units("ppm")
df = composition.comp.pyrochem.normalize_to(norm, units="ppm")
df.pyroplot.REE(unity_line=True, ax=ax, label=composition.name)
ax.legend()
plt.show()
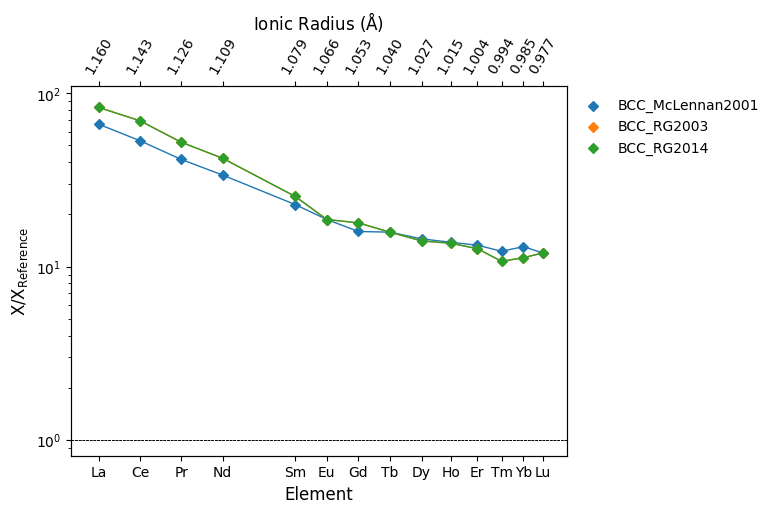
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
Upper Continental Crust
fltr = lambda c: c.reservoir == "UpperContinentalCrust"
compositions = [x for (name, x) in refcomps.items() if fltr(x)]
fig, ax = plt.subplots(1)
for composition in compositions:
composition.set_units("ppm")
df = composition.comp.pyrochem.normalize_to(norm, units="ppm")
df.pyroplot.REE(unity_line=True, ax=ax, label=composition.name)
ax.legend()
plt.show()
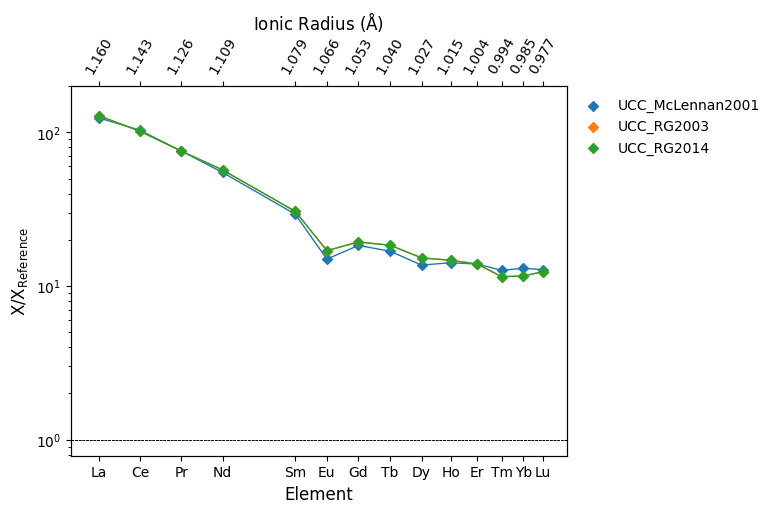
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
Mid-Continental Crust
fltr = lambda c: c.reservoir == "MidContinentalCrust"
compositions = [x for (name, x) in refcomps.items() if fltr(x)]
fig, ax = plt.subplots(1)
for composition in compositions:
composition.set_units("ppm")
df = composition.comp.pyrochem.normalize_to(norm, units="ppm")
df.pyroplot.REE(unity_line=True, ax=ax, label=composition.name)
ax.legend()
plt.show()
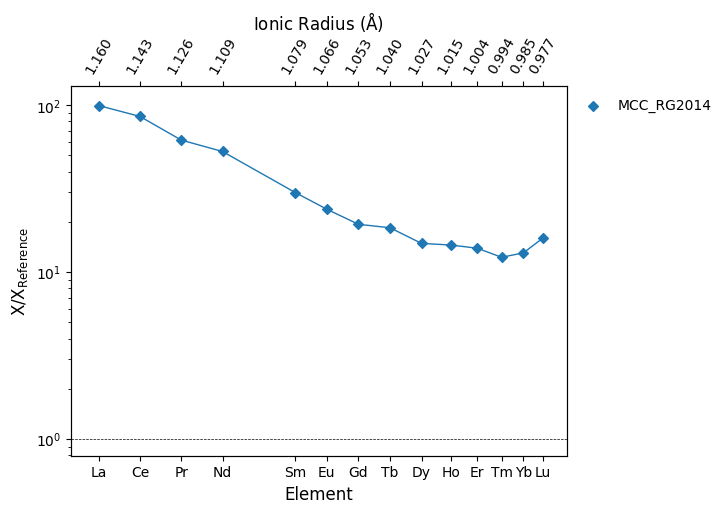
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
Lower Continental Crust
fltr = lambda c: c.reservoir == "LowerContinentalCrust"
compositions = [x for (name, x) in refcomps.items() if fltr(x)]
fig, ax = plt.subplots(1)
for composition in compositions:
composition.set_units("ppm")
df = composition.comp.pyrochem.normalize_to(norm, units="ppm")
df.pyroplot.REE(unity_line=True, ax=ax, label=composition.name)
ax.legend()
plt.show()
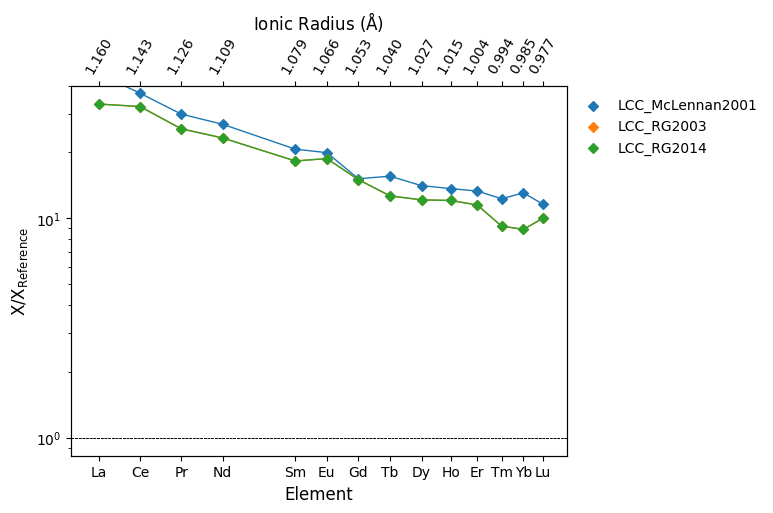
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
Shales
fltr = lambda c: "Shale" in c.reservoir
compositions = [x for (name, x) in refcomps.items() if fltr(x)]
fig, ax = plt.subplots(1)
for composition in compositions:
composition.set_units("ppm")
df = composition.comp.pyrochem.normalize_to(norm, units="ppm")
df.pyroplot.REE(unity_line=True, ax=ax, label=composition.name)
ax.legend()
plt.show()
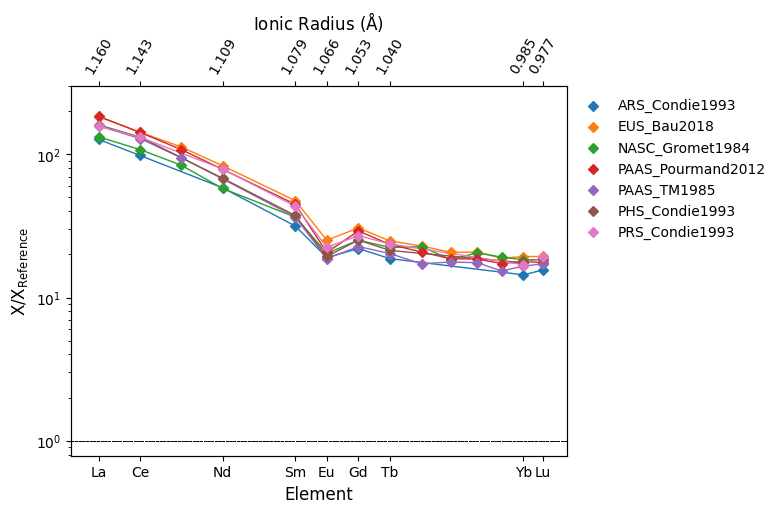
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
/home/docs/checkouts/readthedocs.org/user_builds/pyrolite/checkouts/main/pyrolite/plot/spider.py:276: UserWarning: Attempt to set non-positive ylim on a log-scaled axis will be ignored.
ax.set_ylim(_ymin, _ymax)
Composition List
- NASC_Gromet1984
- NASC_Gromet1984 Model of NorthAmericanShaleComposite from Gromet et al. (1984); Taylor and McLennan (1985); Condie (1993); McLennan (2001).
- EUS_Bau2018
- EUS_Bau2018 Model of EuropeanShale from Bau et al. (2018).
- Chondrite_MS95
- Chondrite_MS95 Model of Chondrite from McDonough & Sun (1995).
- Chondrite_PON
- Chondrite_PON Model of Chondrite from Palme and O'Neill (2014).
- ChondriteREE_ON
- ChondriteREE_ON Model of Chondrite from O'Neill (2016).
- Chondrite_SM89
- Chondrite_SM89 Model of Chondrite from Sun & McDonough (1989).
- E-DMM_WH2005
- E-DMM_WH2005 Model of EnrichedDepletedMORBMantle from Workman & Hart (2005).
- NMORB_Gale2013
- NMORB_Gale2013 Model of NMORB from Gale et al. (2013).
- NMORB_SM89
- NMORB_SM89 Model of NMORB from Sun & McDonough (1989).
- UCC_McLennan2001
- UCC_McLennan2001 Model of UpperContinentalCrust from McLennan (2001).
- UCC_RG2003
- UCC_RG2003 Model of UpperContinentalCrust from Rudnick & Gao (2003).
- UCC_RG2014
- UCC_RG2014 Model of UpperContinentalCrust from Rudnick & Gao (2014).
- MUQ_Kamber2005
- MUQ_Kamber2005 Model of MudFromQueensland from Kamber et al. (2005).
- PHS_Condie1993
- PHS_Condie1993 Model of PhanerozoicCratonicShale from Condie (1993).
- MCC_RG2014
- MCC_RG2014 Model of MidContinentalCrust from Rudnick & Gao (2014).
- BCC_McLennan2001
- BCC_McLennan2001 Model of BulkContinentalCrust from McLennan (2001).
- BCC_RG2003
- BCC_RG2003 Model of BulkContinentalCrust from Rudnick & Gao (2003).
- BCC_RG2014
- BCC_RG2014 Model of BulkContinentalCrust from Rudnick & Gao (2014).
- EMORB_Gale2013
- EMORB_Gale2013 Model of EMORB from Gale et al. (2013).
- EMORB_SM89
- EMORB_SM89 Model of EMORB from Sun & McDonough (1989).
- MORB_Gale2013
- MORB_Gale2013 Model of MORB from Gale et al. (2013).
- OIB_SM89
- OIB_SM89 Model of OIB from Sun & McDonough (1989).
- Pyrolite_MS95
- Pyrolite_MS95 Model of BSE from McDonough & Sun (1995).
- DM_SS2004
- DM_SS2004 Model of DepletedMantle from Salters & Strake (2004).
- DMM_WH2005
- DMM_WH2005 Model of DepletedMORBMantle from Workman & Hart (2005).
- DMORB_Gale2013
- DMORB_Gale2013 Model of DMORB from Gale et al. (2013).
- LCC_McLennan2001
- LCC_McLennan2001 Model of LowerContinentalCrust from McLennan (2001).
- LCC_RG2003
- LCC_RG2003 Model of LowerContinentalCrust from Rudnick & Gao (2003).
- LCC_RG2014
- LCC_RG2014 Model of LowerContinentalCrust from Rudnick & Gao (2014).
- PAAS_Pourmand2012
- PAAS_Pourmand2012 Model of PostArcheanAustralianShale from Pourmand et al. (2012).
- PAAS_TM1985
- PAAS_TM1985 Model of PostArcheanAustralianShale from Taylor & McLennan (1985); McLennan (2001).
- PRS_Condie1993
- PRS_Condie1993 Model of ProterozoicCratonicShale from Condie (1993).
- D-DMM_WH2005
- D-DMM_WH2005 Model of DepletedDepletedMORBMantle from Workman & Hart (2005).
- GLOSS2_P2014
- GLOSS2_P2014 Model of GLOSS from Plank (2014).
- GLOSS_P2014
- GLOSS_P2014 Model of GLOSS from Plank (2014).
- PM_PON
- PM_PON Model of PrimitiveMantle from Palme and O'Neill (2014).
- PM_SM89
- PM_SM89 Model of PrimitiveMantle from Sun & McDonough (1989).
- ARS_Condie1993
- ARS_Condie1993 Model of ArcheanCratonicShale from Condie (1993).
See also
- Examples:
Total running time of the script: (0 minutes 12.379 seconds)